Adding onto our initial discussion on APIs & Webservices, please refer to Part 1 here, we dig deeper into the ingredients that make the API so delicious worthy of usage.
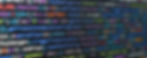
Just like a recipe uses ingredients to make something delicious, an API uses a data format (writing format) to share information between different computer programs. An API, as we talked about earlier, is like a set of rules that two programs can use to communicate with each other. There are two famous data formats that programs can use to share data with each other through an API;
XML
JSON
XML
XML stands for eXtensible Markup Language. World Wide Web Consortium (W3C) organization is the creator of XML.
As we learned from Part 1 of this ongoing article, we know the parts that make up an HTTP. So here, the HTTP Header Line is Content-Type as "application/xml" with the HTTP body as "XML". XML data formatting uses tags "<>" just like HTML for e.g. <button> Click Me! </button> gives the button property to "Click Me!". The fact that the same organization as mentioned above has also created HTML.
These are just the writing style, as for any known language in the world, they follow a set of rules to make it expandable, easy to use & understandable. The same thing happens in the world of programming language, it takes its inference from the real-world language itself, just their talking style is a bit different so is Sanskrit - प्रेम शांतिः आनंदश्च (Love, peace & happiness). Below is a sample code or writing style in XML;
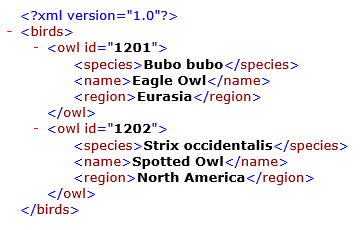
XML Schema & XSD
As I mentioned above that a language follows a specific set of rules, here the XML schema is that set of rules that tells a computer how to read and understand a special kind of text called XML. Just like how you follow rules when playing a game, the computer needs to follow rules to make sure it understands the XML correctly.
These rules tell the computer things like what kinds of information can be in the XML, what names the information can have, and how the different pieces of information are related to each other. It's like a recipe for the computer to understand XML. So, just like you need to follow a recipe to make a cake, the computer needs to follow the XML schema rules to understand the XML correctly.
There are various kinds of XML schema but the one primarily used in APIs is XSD which stands for XML Schema Definition. A sample code for reference is stated below;

JSON
JSON stands for JavaScript Object Notation. It was created by a single developer Mr. Douglas Crockford, unlike XML which was created by 11 people with support from other 150.
In JSON, the HTTP Header Line is Content-Type as "application/json" with the HTTP body as "JSON". JSON data formatting uses key-value pairs. An example is - "Size": "Small", as simple as that. Below is the sample code of JSON for further deliberation;
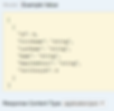
If you intend to view JSON files in the google chrome browser use the JSON extension e.g. JSONView but in the Firefox browser, you can directly view JSON files.
JSON is small, lightweight, and simple to understand, hence when you have millions of transactions taking place you need a language like this to take up the load. So it's the most preferred format in APIs for the internet or web services. It does have a schema but the majority of developers don't use it, they just follow the simple JSON format.
Data structures
Data structures are how data gets stored and organized in JavaScript. There are 4 basic data structures:
Key/value pairs: Like text field, text area, phone number, radio buttons, and more.
Objects: An object is a type of data structure that represents a single, self-contained entity. The object acts as a container for the characteristics of that entity. These characteristics store as key/value pairs in the object. The value of one of those key/value pairs can itself be an object or an array. JSON uses curly braces ({...}) to denote objects.
Arrays: It's a way to group related elements. Arrays can contain values, objects, and even other arrays. A key feature of the array data structure is that arrays use indexing. Indexing is a way to assign an index number to each element in the array. This index gives each element a specific position in the array, for easy reference to a value. In arrays, the index numbering starts at 0.
Tables

XML Vs JSON
Following is a comparison of both the language for HTTP / API uses;
| XML | JSON |
Powerful | Yes | No |
Simple | No | Yes |
Developed | 1997 | 2001 |
Popularity | Down | Up |
Discussing the popularity and trends, you can clearly see that JSON is preferred over XML. And it's been stated that in the present time if you are new to web services you probably won't see XML usage anymore, either JSON or any other new data format.
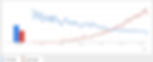
Now we will discuss the two most important ways to create HTTP Requests & Responses i.e. SOAP & REST.
Before we move further to understand how they work, I intend to show the emerging trends for both the method;

So here we can clearly see that the SOAP method is kind of stagnant in its usage whereas the REST method has skyrocketed and currently almost all the applications in web services are using this method only.
SOAP
SOAP stands for Simple Object Access Protocol, it says simple but it's not that simple. The object is the data that is to be transferred and access protocol is the rules on how it should be done; It's the rules to form HTTP Request/Response. SOAP API uses Web Services Description Language - WSDL to format the API which is in XML. As noted in the previous article the 4 HTTP Request Parts in relation to SOAP is as follows;
Start Line: POST WSDL HTTP Version
Header Line: Content-Type: text/xml
Blank Line
Body: XML envelope formed using WSDL
As you can see all the XML & WSDL, you are correct in your inference that it was created by W3C (who also created XML & HTML). Below is an example of a sample body code;

So, in layman's terms, if one program wants to talk to another program using SOAP API, it sends a message in XML format. The other program receives the message and uses SOAP API to understand it and respond back in XML format. This way, they can share information and work together even if they're using different programming languages.
Currently, I can rarely find any application which exclusively uses SOAP APIs, but below are a few famous real-life web applications that offer SOAP APIs;
Salesforce: It uses SOAP API to integrate with other applications and services, such as marketing automation software or accounting software.
PayPal: Uses SOAP API to provide developers with a way to integrate PayPal functionality into their own applications, such as online stores or invoicing systems.
Amazon Web Services (AWS): Many of its services like storage, computing power, and databases are offered in SOAP API interfaces to allow developers to interact with them programmatically.
FedEx: It provides SOAP API to developers with a way to integrate FedEx shipping functionality into their own applications, such as e-commerce platforms or shipping management software.
REST
Below is the snapshot of the Evolution of API, which give you a context of how everything has come to date;
Time | API Method |
1980 | SUN RPC |
1998 | XML-RPC |
1999 | SOAP |
2000 | REST |
So as you have observed in image 6, you can rightly assume that REST API is the way forward for web services. Below is an explanation to give a small context for the reason behind it;
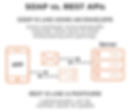
REST API is lightweight in the context that it uses the method in its start line itself (you do have "GET" in SOAP but it does nothing much, Ref.: HTTP: General structure of the start line - In part 1 of this article) and further cache the data searched similarly like cookies to save inputs for faster execution.
REST stands for Representational State Transfer and is definitely used to complete a Web Service (API) using HTTP, that's what we are discussing all along. The great thing about this method is that it has no rules unlike SOAP, that's what we all love freedom. And amazingly it was developed by single creator Roy Fielding.
As noted previously the 4 HTTP Request Parts in relation to REST are as follows;
Start Line: All methods (GET, POST, PUT, DELETE, etc)
Header Line: All i.e. any header
Blank Line
Body: Any i.e. JSON, XML, images, HTML web pages, etc.
There are two most important concepts which must be clear now to make sense of why almost all applications on the web prefer REST over SOAP. To further provide a build on that context, the method that is used in HTTP are many but the main ones are stated below in conjunction with the database method of editing table as CRUD operations;
POST > Create
GET > Read
PUT > Update
DELETE > Delete
Besides "Cache" in the REST APIs used to retrieve data from local memory rather than from the web server; which makes the entire platform lightweight, faster & powerful.
Almost all contemporary real-life applications use REST API, here are a few examples:
Social Media Platforms: Social media platforms like Twitter, Facebook, and Instagram use REST API to allow third-party developers to access their data and integrate their platforms with other applications. For example, a developer can use the Twitter REST API to create a social media monitoring tool that tracks mentions of a particular keyword or hashtag.
E-commerce Websites: E-commerce websites like Amazon and Flipkart use REST API to allow developers to access their product catalogs and order information. This allows developers to create custom applications that integrate with these websites, such as price comparison tools or inventory management systems.
Weather Applications: Weather applications like AccuWeather and Weather Underground use REST API to provide weather data to other applications. For example, a developer can use the AccuWeather REST API to create a weather app that provides real-time weather updates and alerts.
Banking Applications: Banking applications like PhonePe and Razorpay use REST API to allow developers to access their payment processing services. This allows developers to create custom applications that accept payments, manage transactions, and provide detailed financial reporting.
Overall, REST API is widely used in modern software development to create flexible and scalable applications that can communicate and share data with other applications.
-----
All the related articles for "The API, the Webservice & a short explanatory Story for the non-programmer"
-----
*Source:
Image 0: Programming codes - Unsplash
Image 1: https://help.seagullscientific.com/2019/en/Content/DB_Types_XMLStructureOverview.htm
Image 2: https://study.com/academy/lesson/what-is-xsd-examples-tutorial.html
Image 3: https://stackoverflow.com/questions/45922832/c-sharp-library-for-converting-json-schema-to-sample-json
Image 5: https://www.toptal.com/web/json-vs-xml-part-1
Image 6: https://www.researchgate.net/figure/Trends-of-XML-and-JSON-API-in-Google-searches_fig2_296702598
Image 8: https://jelvix.com/blog/rest-vs-soap
*Reference